Ma kasutasin yandex api ja rick and morty api.
https://denissgorjunov22.thkit.ee/RickAndMortyAPI/index.html#
Kasutaja saab Rick ja Morty API-st juhusliku tegelase ja vaadata selle tegelase kohta üksikasjalikku teavet. Kaardil saab kasutaja näha antud aadressile seatud markerit.
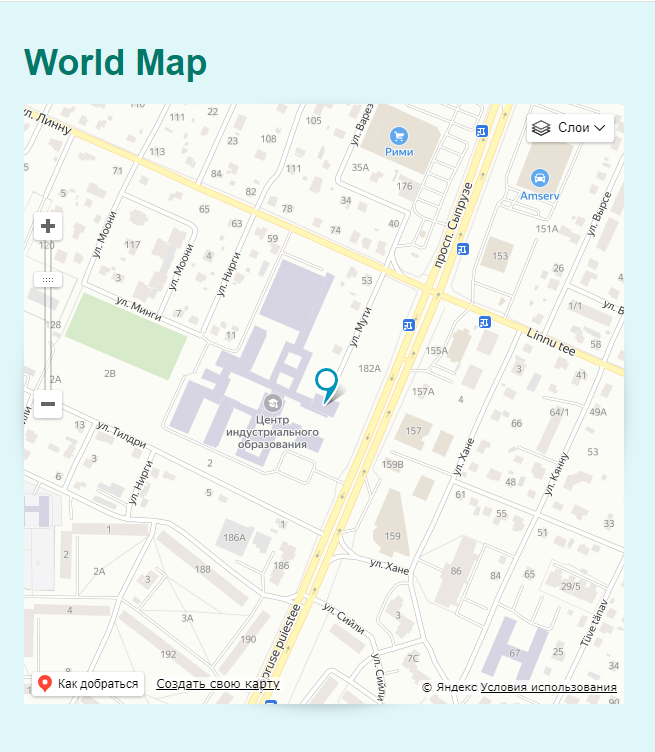
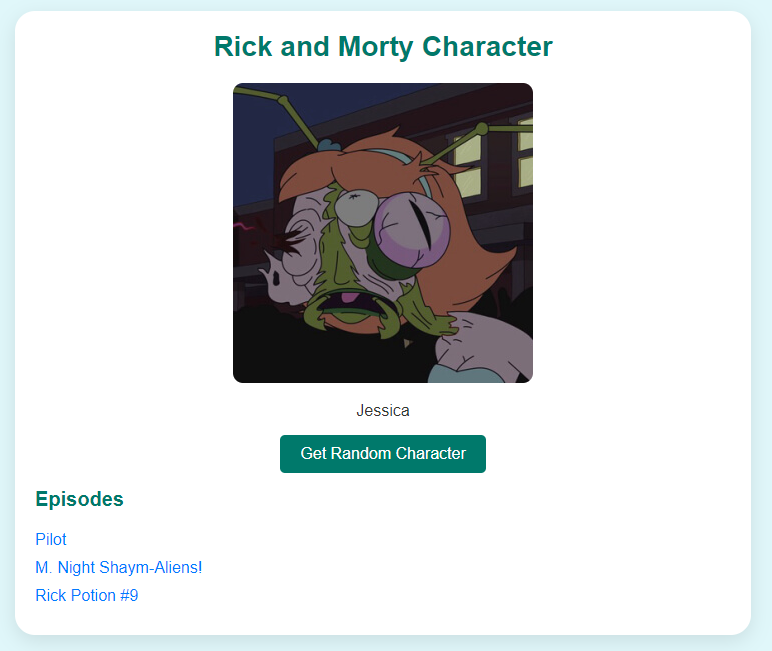

Lühikesed juhised projekti alustamiseks
Looge HTML-faili:
<!DOCTYPE html>
<html lang="ru">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://api-maps.yandex.ru/2.1/?lang=ru_RU&apikey=c05f1a8c-70a4-4f96-8dbb-2321dfc2f203"></script>
<script src="https://yandex.st/jquery/1.6.4/jquery.min.js"></script>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="container">
<h1>World Map</h1>
<div id="map"></div>
<p>© Deniss Gorjunov TARpv22</p>
</div>
<div id="rick-morty-container">
<h2>Rick and Morty Character</h2>
<div id="character-info">
<img id="character-image" src="" alt="Character Image">
<p id="character-name">Character Name</p>
</div>
<button id="random-character-btn" class="button-animate">Get Random Character</button>
<div id="episodes">
<h3>Episodes</h3>
<ul id="episode-list"></ul>
</div>
<div id="episode-details">
<h4 id="episode-title">Episode Title</h4>
<p id="episode-air-date">Air Date: </p>
<p id="episode-summary">Episode Description: </p>
</div>
</div>
<script src="main.js"></script>
<script src="map.js"></script>
</body>
</html>
Looge fail index.html ja lisage sellele veebilehe struktuur koos konteineritega kaardi ja Rick and Morty API andmete jaoks.
Ühendage CSS:
Looge style.css fail, et lehte stiliseerida (näiteks lisada stiilid kaardile, tegelaste ja episoodi infoblokkidele).
* {
box-sizing: border-box;
margin: 0;
padding: 0;
}
html, body {
width: 100%;
height: 100%;
font-family: 'Arial', sans-serif;
background-color: #e0f7fa;
display: flex;
justify-content: space-between;
align-items: flex-start;
padding: 20px;
color: #333;
}
h1, h2, h3 {
margin-bottom: 20px;
color: #00796b;
}
h1 {
font-size: 36px;
}
h2 {
font-size: 28px;
}
h3 {
font-size: 20px;
}
#container {
display: flex;
flex-direction: column;
align-items: flex-start;
margin-right: 20px;
width: 50%;
border-radius: 50%;
}
#map {
width: 100%;
height: 50vw;
max-width: 600px;
max-height: 600px;
border: none;
border-radius: 50%;
box-shadow: 0px 4px 12px rgba(0, 0, 0, 0.2);
}
@media (max-width: 600px) {
#map {
width: 50%;
height: 50vw;
border-radius: 100%;
}
}
#rick-morty-container {
width: 40%;
background-color: white;
border-radius: 20px;
padding: 20px;
box-shadow: 0px 6px 20px rgba(0, 0, 0, 0.1);
display: flex;
flex-direction: column;
align-items: center;
text-align: center;
}
#rick-morty-container img {
width: 100%;
max-width: 300px;
border-radius: 10px;
margin-bottom: 15px;
transition: transform 0.3s ease;
}
#rick-morty-container img:hover {
transform: scale(1.05);
}
#rick-morty-container button {
margin-top: 15px;
padding: 10px 20px;
font-size: 16px;
background-color: #00796b;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
#rick-morty-container button:hover {
background-color: #004d40;
}
#episodes {
margin-top: 15px;
text-align: left;
width: 100%;
}
#episodes ul {
list-style: none;
padding: 0;
}
#episodes li {
margin-bottom: 10px;
font-size: 16px;
}
#episodes a {
text-decoration: none;
color: #007bff;
transition: color 0.2s ease;
}
#episodes a:hover {
color: #0056b3;
}
#episode-details {
margin-top: 20px;
background-color: #f1f8e9;
padding: 15px;
border-radius: 10px;
box-shadow: 0px 4px 10px rgba(0, 0, 0, 0.1);
display: none;
text-align: left;
}
#episode-title {
font-size: 18px;
font-weight: bold;
}
#episode-air-date, #episode-summary {
margin-top: 10px;
}
.button-animate {
transition: all 0.3s ease-in-out;
}
.button-animate:hover {
transform: scale(1.05);
}
Lisage JavaScript:
Looge fail main.js funktsioonidega, et töötada Yandex.Maps API ja Rick and Morty APIga:
Ühendage Yandex.Maps ja konfigureerige nende initsialiseerimine.
Lisage kood, et saada Rick ja Morty API-st juhuslik tegelane ja kuvada teave.
Ühendage Yandex.Maps API:
Registreeruge Yandex API-le ja hankige API võti.
Sisestage
main.js
const API_URL = 'https://rickandmortyapi.com/api/character/';
const randomCharacterBtn = document.getElementById('random-character-btn');
const characterImage = document.getElementById('character-image');
const characterName = document.getElementById('character-name');
const episodeList = document.getElementById('episode-list');
const episodeDetails = document.getElementById('episode-details');
const episodeTitle = document.getElementById('episode-title');
const episodeAirDate = document.getElementById('episode-air-date');
const episodeSummary = document.getElementById('episode-summary');
const characterLocation = document.createElement('p');
episodeDetails.appendChild(characterLocation);
async function getRandomCharacter() {
try {
const randomId = Math.floor(Math.random() * 671) + 1;
const response = await fetch(`${API_URL}${randomId}`);
const character = await response.json();
displayCharacter(character);
getCharacterEpisodes(character.episode);
displayLocation(character);
} catch (error) {
console.error('Error fetching character:', error);
}
}
function displayCharacter(character) {
characterImage.src = character.image;
characterName.textContent = character.name;
}
async function getCharacterEpisodes(episodes) {
episodeList.innerHTML = '';
for (let episodeUrl of episodes) {
const response = await fetch(episodeUrl);
const episode = await response.json();
const episodeItem = document.createElement('li');
episodeItem.innerHTML = `<a href="#" onclick="showEpisodeDetails('${episode.url}')">${episode.name}</a>`;
episodeList.appendChild(episodeItem);
}
}
function displayLocation(character) {
const origin = character.origin.name !== 'unknown' ? character.origin.name : 'Unknown origin';
const location = character.location.name !== 'unknown' ? character.location.name : 'Unknown location';
characterLocation.innerHTML = `
<strong>Origin:</strong> ${origin} <br>
<strong>Current location:</strong> ${location}
`;
}
async function showEpisodeDetails(episodeUrl) {
try {
const response = await fetch(episodeUrl);
const episode = await response.json();
episodeTitle.textContent = episode.name;
episodeAirDate.textContent = `Air Date: ${episode.air_date}`;
episodeSummary.textContent = `Episode Description: This episode aired on ${episode.air_date}. It is episode ${episode.episode} in the series.`;
episodeDetails.style.display = 'block';
} catch (error) {
console.error('Error fetching episode details:', error);
}
}
randomCharacterBtn.addEventListener('click', getRandomCharacter);
getRandomCharacter();
map.js
ymaps.ready(init);
function init() {
var myMap = new ymaps.Map("map", {
center: [59.3594, 27.4216],
zoom: 10,
controls: ['zoomControl', 'typeSelector']
});
ymaps.geocode('Sõpruse pst 182, Estonia').then(function (res) {
var firstGeoObject = res.geoObjects.get(0);
if (!firstGeoObject) {
console.error('Адрес не найден!');
return;
}
var coords = firstGeoObject.geometry.getCoordinates();
var myPlacemark = new ymaps.Placemark(coords, {
balloonContent: 'Sõpruse pst 182'
}, {
preset: 'islands#icon',
iconColor: '#0095b6' // Цвет маркера
});
myMap.geoObjects.add(myPlacemark);
myMap.setCenter(coords, 16);
}).catch(function (error) {
console.error('Ошибка при геокодировании:', error);
});
}
KML is a file format used to display geographic data in an Earth browser such as Google Earth. KML uses a tag-based structure with nested elements and attributes and is based on the XML standard. All tags are case-sensitive and must appear exactly as they are listed in the KML Reference. The Reference indicates which tags are optional. Within a given element, tags must appear in the order shown in the Reference.
xml file
<?xml version="1.0" encoding="UTF-8"?>
<kml xmlns="http://www.opengis.net/kml/2.2">
<Document>
<name>Example Map</name>
<description>This is a simple example of a KML file</description>
<!-- Style for the placemark -->
<Style id="exampleStyle">
<IconStyle>
<color>ff0000ff</color> <!-- Blue color in ABGR format -->
<Icon>
<href>http://maps.google.com/mapfiles/kml/shapes/placemark_circle.png</href>
</Icon>
</IconStyle>
</Style>
<!-- A placemark for a specific location -->
<Placemark>
<name>Sõpruse pst 182, Estonia</name>
<description>This is the location on Sõpruse pst in Estonia.</description>
<styleUrl>#exampleStyle</styleUrl>
<Point>
<coordinates>27.4216,59.3594,0</coordinates>
</Point>
</Placemark>
<!-- A polygon example -->
<Placemark>
<name>Example Polygon</name>
<description>A simple polygon</description>
<Polygon>
<outerBoundaryIs>
<LinearRing>
<coordinates>
27.4216,59.3594,0
27.4230,59.3600,0
27.4245,59.3595,0
27.4216,59.3594,0
</coordinates>
</LinearRing>
</outerBoundaryIs>
</Polygon>
</Placemark>
</Document>
</kml>